I updated the HTML panel with SVG graphics to replace the old table grids.
Author: tim
Machine Learning Models
History: Kings and Generals
Recommended Channel: [Kings and Generals]
Mixer Two-Way Communication with Custom Controls and Game Clients
The Mixer streaming platform supports custom widget overlays over the video streams. Those widgets support [two-way communication].
Emotiv MN8
Let us hope the SDK and raw EEG access is free with the new [Emotiv MN8] product.
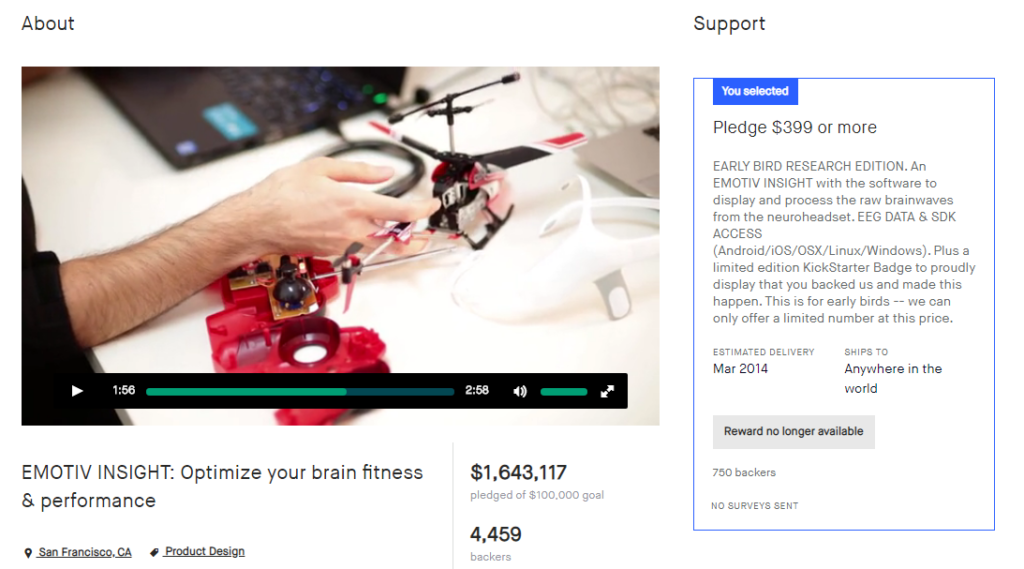
Chroma Minecraft Mod Weather
Prusa MK3S Firmware Update 3.8.0
Minecraft Mod – More Chroma Effects
Thermal Runaway
I’ve been printing with 265C on the first layer to avoid the print slipping off the bed, but when I do that sometimes I get a Thermal Runaway
error.
Unreal Film Jam – September 5 – October 19
[Unreal Film Jam] Create a short film for a chance to win $5000.
RESOURCES
UNREAL ONLINE LEARNING
YOUTUBE TECH TALKS
- Cinematic Lighting in Unreal Engine
- Enabling a Look Development Workflow for UE4: From Shoot to Final LookDev Scene
- Lighting with Unreal Engine Masterclass
- Making the Most of Animation Blueprints
- New Animation Features in UE4
- The Virtual Production Field Guide: a new resource for filmmakers
- Volumetric Fog and Lighting in Unreal Engine
Mixer Chroma Game Client – SVG Update – Apex Legends
Extruder motor visualizer
Prusa MK3S Hotend Broken
Normally the hotend looks like this… (This was the old one that I replaced because it leaked.)
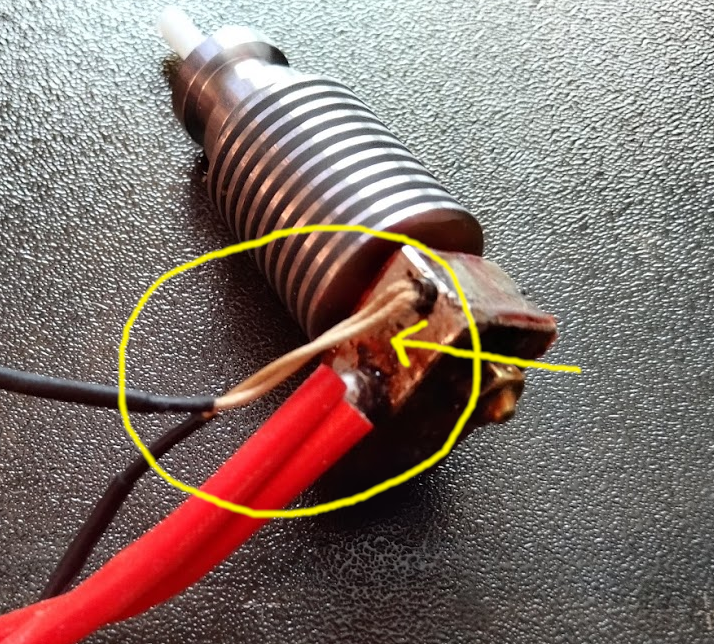
I was in starting a 3d print when the thermistor wire broke on the hotend.
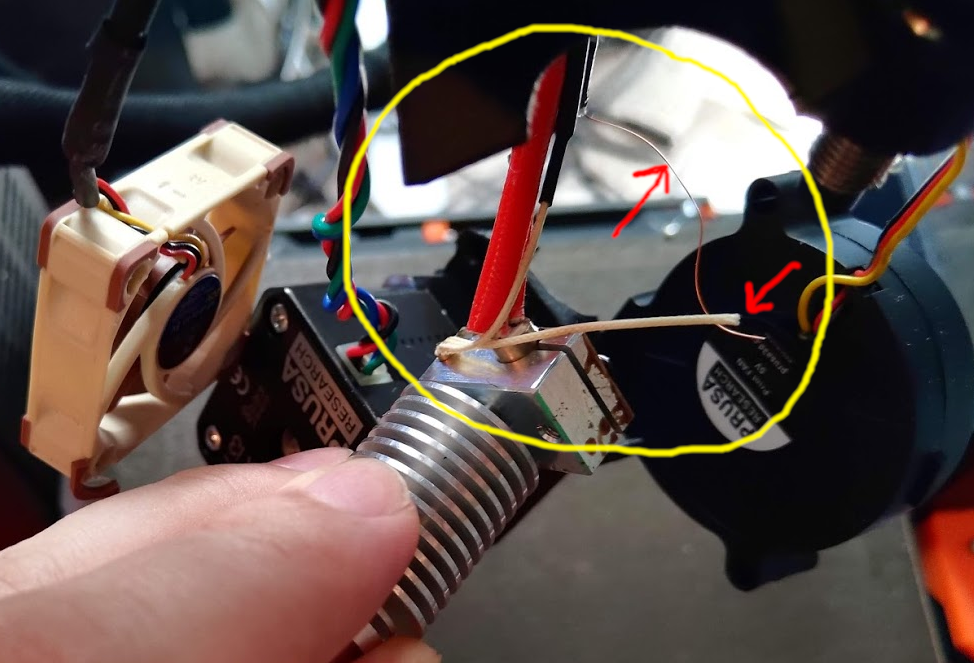
Luckily the hotend is still [In Stock].

Building Animatronic Robot Heads
Mixer Chroma Game Client – SVG Update
Razer launch their Gears 5 branded Xbox and PC range
Mixer – SVG Content Document
I’m having an issue with Mixer not being able to access the SVG DOM. I made a repro project.
Adafruit HalloWing M4 Express
Chroma Minecraft Mod – Chroma Effects
Unity Word Detection – Version 1.12
Unreal 4.23 Released
Platforms
- HTML5 platform support will be migrated to GitHub as a community-supported [Platform Extension] and no longer officially supported by Epic in upcoming releases.
Minecraft Chroma Mod
I created a MinecraftForge mod the detects in game events and plays Chroma animations. [MinecraftChromaMod]
Requires [JDK 1.8]
Rocksmith – Customs Forge
The [CustomsForge] tool allows custom songs to be added to the guitar game “RockSmith”.